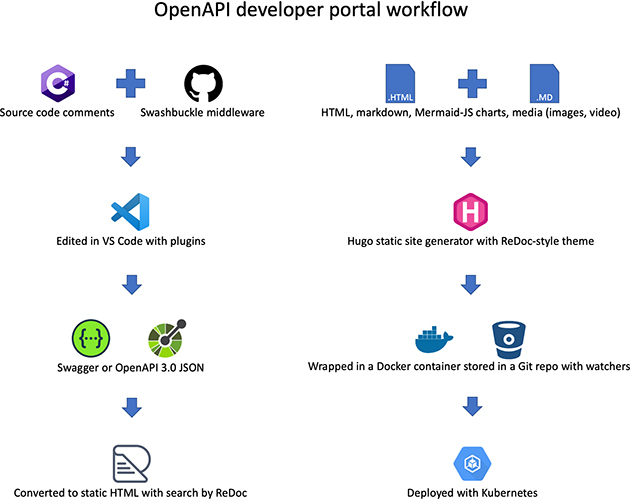
Published on 27 January 2022 by Andrew Owen (4 minutes)
In this three part series, I’ll outline how to create a fully featured dev portal for your Swagger or OpenAPI 3.0 content without spending a dime. You can read part one here and part three here.
When I wrote the original version of this guide, I was an API writer creating docs for an event-driven e-commerce solution. Event driven architecture (EDA) is something I’ll talk more about in a future article. Development was done primarily in .NET and API docs were generated using Swashbuckle (also a good topic for a future article). This is known as the code first or bottom up approach. The more your code grows, the harder it is to maintain consistent APIs with this approach.
In my view, you should create your API specification and code against that. But for the purposes of this guide, I’ll assume that you don’t have any say in that, and you just have to get the API docs into the hands of developers. You may not even have an API schema, in which case you should create one in Postman, based on whatever API documentation you have available to you.
If you’re using windows and writing API docs directly in the code, you may be using Visual Studio. If you’re not using Windows, then the next best thing is Visual Studio Code.
Before you set up your dev environment, ensure you have installed the CLI tools.
development
where you want to store your local repositories.development
folder.git clone
and paste the HTTP address to complete the line.This assumes you have a working .NET dev environment and have correctly configured Swashbuckle:
dotnet build
.dotnet run
.http://127.0.0.1:5000
./swagger/v1/swagger.json
link to download the JSON file.Some recommended plug-ins for Visual Studio Code include:
.vscode/
to the .gitignore
file to any repository you intend to use tasks with.Enter: redoc-cli bundle <filename>.json
.
This file should be placed inside the .vscode
folder in the Git repository and .vscode
added to the .gitignore
file.
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"command": "dotnet",
"type": "process",
"args": [
"build",
"${workspaceFolder}/src/Dev.Example.Com.Myproject/Devg.Example.Com.Myproject.csproj"
],
"problemMatcher": "$msCompile"
},
{
"label": "Swagger",
"type": "shell",
"command": ".vscode/swagger.sh",
"windows": {
"command": ".vscode\\swagger.cmd"
},
"presentation": {
"reveal": "always",
"panel": "new"
}
},
{
"label": "ReDoc",
"type": "shell",
"command": ".vscode/redoc.sh",
"windows": {
"command": ".vscode\\redoc.cmd"
},
"presentation": {
"reveal": "always",
"panel": "new"
},
"problemMatcher": []
},
{
"label": "Build API Docs",
"dependsOn": ["Swagger
- ReDoc"],
"group": {
"kind": "build",
"isDefault": true
},
}
]
}
cd ~/
rm redoc.jsonrm redoc-static.html
# wait for server to start
echo waiting for service to start
sleep 10
curl -o redoc.json http://localhost:62512/swagger/v1/swagger.json
redoc-cli bundle redoc.json
open -a "Google Chrome" redoc-static.html
export CONSUL=[http://consul.dev.example.com](http://consul.dev.example.com "http://consul.dev.example.com")
cd src
dotnet build --source [http://dev.example.com/myproject/](http://dev.example.com/myproject/ "http://dev.example.com/myproject/")
cd Dev.Example.Com.Myproject
dotnet run --urls=http://localhost:62512
cd ~/
del redoc.json
del redoc-static.html
# wait for server to start
echo waiting for service to start
sleep 10
curl -o redoc.json
set CONSUL=http://consul.dev.example.com
cd src
dotnet build --source http://dev.example.com/myproject/
cd Dev.Example.Com.Myproject
dotnet run --urls=http://localhost:62512