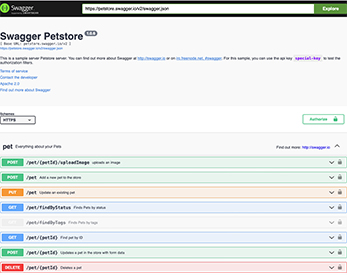
Published on 20 January 2022 by Andrew Owen (9 minutes)
In this three part series, I’ll outline how to create a fully featured dev portal for your Swagger or OpenAPI 3.0 content without spending a dime. You can read part two here and part three here.
A dev portal is just a website that presents API (and other) docs to developers. It can be as simple as a static web page or as complex as you want to make it. In this series, we’ll make use of the following back-end technologies:
In addition, you’ll inevitably spend a fair amount of time using a browser. For better or worse, Chrome is the new standard.
Hugo is a type of web server known as a static site generator (SSG). It’s lightweight and fast and can serve dynamic and static content. You can test content locally before deploying it. For OpenAPI content, we’ll use the command line version of ReDoc to convert Swagger JSON files to a static HTML page that’s served by Hugo.
/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
git
and follow the instructions to install Xcode.brew install hugo
.brew install nodejs
.
ReDoc renders an OpenAPI file into a static HTML page. From the Terminal, enter npm install -g redoc-cli@0.13.2
. You can leave off the @<version_number>
, but I’ve found npm doesn’t always get the latest version if you do. Ensure you’re using version 0.9.8 or later:redoc-cli --version\
.npm install swagger-spec-to-pdf
.scoop install git
.scoop install hugo
.scoop install nodejs
.npm install -g redoc-cli0.13.2
. You can leave off the @
<version number>
, but I’ve found npm doesn’t always get the latest version if you do. Ensure you’re using version 0.9.8 or later:redoc-cli --version
.npm install swagger-spec-to-pdf
.scoop install curl
.Navigate to the folder where you have cloned the Git repository.
hugo server
.Paste the following text after the <head>
tag:
<!-- Global site tag (gtag.js) - Google Analytics --><script async src="https://www.googletagmanager.com/gtag/js? id=<yourID>"></script>
If you’re generating comments from code, you’ll be working directly in the software repository. Typically, this is a Git repository. You should familiarize yourself with the processes for creating branches, creating pull requests, resolving conflicts and merging changes. There are a number of commercial hosting options including GitHub, GitLab and Bitbucket. Here we’ll look at Bitbucket.
~/development/
.git clone
and paste the path you copied from Chrome. This creates a local copy of the repository.feature/apidocs-
<api class name>
) and select the branch to base it on. Example: development
You can now make your changes.
You’ll receive email notifications when the status of the pull request changes. Example: When the change is approved.
git pull origin master
.git commit -am "
<your commit message>
"
git push
In Chrome, navigate to the pull request and click Merge.
After the change is successfully merged, from the left icon menu, click Branches.
Locate the branch you were working in and from the Actions menu, select Delete
branch.
Click Delete to confirm your action.
While not directly related to APIs, you may on occasion have to modify text contained in a file in a Git repository stored in Bitbucket. You must have a Bitbucket account to edit the files.
feature/uitext
.When your pull request has been reviewed and there has been at least one successful build, providing that there are no merge conflicts, you can merge your change.
If the merge was successful, you should now delete your branch.
Containers are an operating system-level virtualization technology. Sun Microsystems’ Solaris Zones were an early implementation in 2004. But the technology was popularized by Docker containers, release in 2013, and Kubernetes container management, released the following year.
You don’t need containerization if you’re using a hosting solution such as Netlify. However, if you’re hosting your own site, then you should at least consider using containers.
If you deploy your dev portal in a Docker container, you can try it locally before you build your automation tool chain with Docker Desktop.
After installation, you can run Docker images locally. Example: docker run -d --name rabbitmq -p 15672:15672 -p 5672:5672 bitnami/rabbitmq:latest
The easiest way to deploy the Hugo static site is in a Docker image, as defined by a Dockerfile:
FROM
defines the base image (in the example Alpine, a lightweight Linux distribution).COPY
copies files and folders to the Docker image.ARG
specifies arguments for the Docker build command.RUN
executes commands.EXPOSE
informs a user about the ports used.CMD
specifies the component and its arguments to be used by the image.Here’s an example:
FROM alpine:3.8 as runner
COPY . .
ARG HUGO_VERSION=0.80.0
RUN apk --no-cache add \
curl \
git \
&& curl -SL https://github.com/gohugoio/hugo/releases/download/v
${HUGO_VERSION}/hugo_${HUGO_VERSION}_Linux-64bit.tar.gz \
-o /tmp/hugo.tar.gz \
&& tar -xzf /tmp/hugo.tar.gz -C /tmp \
&& mv /tmp/hugo /usr/local/bin/ \
&& apk del curl \
&& rm -rf /tmp/*
EXPOSE 80
CMD hugo --renderToDisk=true --watch=true --port=80 --bind="0.0.0.0" --
baseURL="${VIRTUAL_HOST}" server
Some APIs may require middleware, for example a local RabbitMQ instance. It can be convenient to have Docker start the middleware when you log in. For example, on macOS:
Open the Automator application.
Select the Application type and click Choose.
In the Actions menu, select Utilities > Run Shell Script.
In the Run Shell Script section, select /bin/bash
from the Shell menu.
In the box, enter:
cd /usr/local/bin
while (! ./docker stats --no-stream ); do
sleep 10
done
./docker start rabbitmq
From the File menu, click Save.
Navigate to the Applications folder (or your user Applications folder).
Enter StartRabbit in the Save As box and click Save.
Open System Preferences and click Users & Groups.
Select your user and click Login Items.
Click Add (+).
Navigate to the location where you saved the StartRabbit application and select it.
Click Add.
Close System Preferences.
The script will run the next time you log in. The path has to be changed because /usr/local/bin
isn’t part of the path for startup scripts. The script waits for the Docker daemon to start before starting the RabbitMQ container. It does this by querying Docker until it gets a response. A spinning cog is displayed in the right menu while the script is running.
If you’re deploying more than one Docker instance, that’s the perfect time to start using Kubernetes.
You should automate your deployments so that when your code changes, your API docs are updated. When software is developed using continuous integration and deployment, it helps if the docs are deployed that way too. A popular open source solutions for this is Jenkins. But if you’re using GitHub, you can use GitHub Actions:
name: Build ReDoc HTML
on:
push:
paths:
- 'static/apis/**.yaml'
workflow_dispatch:
jobs:
redoc:
runs-on: ubuntu-latest
permissions:
contents: write
steps:
- uses: actions/checkout@v4
- name: Create local changes
run: |
npm i -g redoc-cli
redoc-cli build static/apis/swagger.yaml -o static/apis/swagger.html
- name: Commit files
run: |
git config --local user.email "github-actions@users.noreply.github.com"
git config --local user.name "github-actions"
git add .
git commit -a -m "Add changes"
- name: Push changes
run: git push origin main