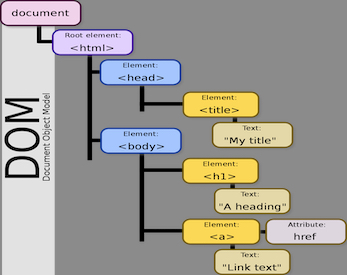
Published on 2 February 2023 by Andrew Owen (4 minutes)
This week, I had a look at the Document Object Model (DOM) for the first time since graduating from my computer science degree over a decade ago. I can’t remember the last time I created a web page by hand in HTML. I’d much rather use a static site generator with a pre-defined theme and only change things if absolutely necessary. I’m also open to other solutions for automatically generating pages. But sometimes you want to change something on the page, and often it’s something that you can’t access directly from the settings. However, sometimes the settings allow you to include snippets of JavaScript, for example to support analytics. And this can provide a workaround.
According to Mozilla: “The DOM is the data representation of the objects that comprise the structure and content of a document on the web.” It provides a guide that introduces the DOM, explains how it represents an HTML document in memory and how to use APIs to create web content and applications. The history of the DOM is tied up in the Microsoft Netscape browser war. The last release was DOM4 in 2015, but it’s now managed as a living document by the Web Hypertext Application Technology Working Group (WHATWG). The group was created in response to the decision of the World Wide Web Consortium (W3C) to ditch HTML in favor of XHTML. Instead, it created HTML5.
But that’s enough web history for today. Back to replacing content on a web page. For example, say you want to override the default <footer> element on a page. The following JavaScript will do the trick:
window.onload = function () {
document.getElementsByTagName("footer")[0].outerHTML = "<footer>...</footer>";
};
You may need to enclose it in <script>
tags, or save it as a .js
file and upload that, depending on what tools you’re using. Here’s how it works:
window.onload
makes the JavaScript run when the page is loaded.document.getElementsBy
gets an element of DOM by some reference, in this case TagName
. It’s also common to reference elements by their ID
if it’s known.("footer")[0]
looks for a match for a <footer>
tag, and [0]
looks for the first instance (programmers start counting at zero)..outerHTML
means that the tag itself will be replaced. For example, you could change a footer into a regular paragraph. If you want to select only what’s between the opening and closing tags, you can use .innerHTML
instead.= ""
specifies what will replace the selected element. If you use quotes ( "
) within the string, then you must escape them ( /"
).And that’s all there is to it. The Mozilla document I mentioned earlier is an excellent reference if you’re looking to do something a bit more complicated than this. When I first wrote this, I promised that if I found that I needed to do anything else DOM related, I’d come back and update this article. Well, I needed to change every element on a page with the class external-top-nav-link to make its target _self. Here’s how I did it:
window.onload = function () {
var className = document.getElementsByClassName('external-top-nav-link');
for (var index = 0; index < className.length; index++) {
className[index].target = "_self";
};
};
I defined the variable className and used the length property to set up a for loop that iterates over all the instances on the page where the class name is external-top-nav-link.
The next thing I had a go at was adding an element to every page to enable the user to select a language for the site. This is based on the assumption that the path will be the same, except for the language folder.
window.onload = function () {
var div = document.createElement('div');
div.className = 'langSelect';
div.id = 'op';
div.style.cssText = 'position: absolute; z-index: 999; height: 16px; width: 16px; top:70px';
div.innerHTML = "<select id=\"mySelect\" onchange=\"toggleLang()\"><option selected disabled>Language</option><option value=\"en/\">English</option><option value=\"fr/\">français</option><option value=\"de/\">Deutsch</option></select>";
document.body.appendChild(div);
};
function toggleLang() {
window.location.assign("../" + document.getElementById("mySelect").value + window.location.pathname.substring(window.location.pathname.lastIndexOf('/') + 1))
}
The function that runs when the page is loaded inserts an element into the page with the following contents:
<div class="langSelect" id="op" style="position: absolute; z-index: 999; height: 16px; width: 16px; top: 70px;">
<select id="mySelect" onchange="toggleLang()">
<option selected="" disabled="">Language</option>
<option value="en/">English</option>
<option value="fr/">français</option>
<option value="de/">Deutsch</option>
</select>
</div>
When the user makes a selection, the toggleLang() function is called and the current language path replaced with the one held in value.